How To Turn Log Splitter Into Filter Crusher
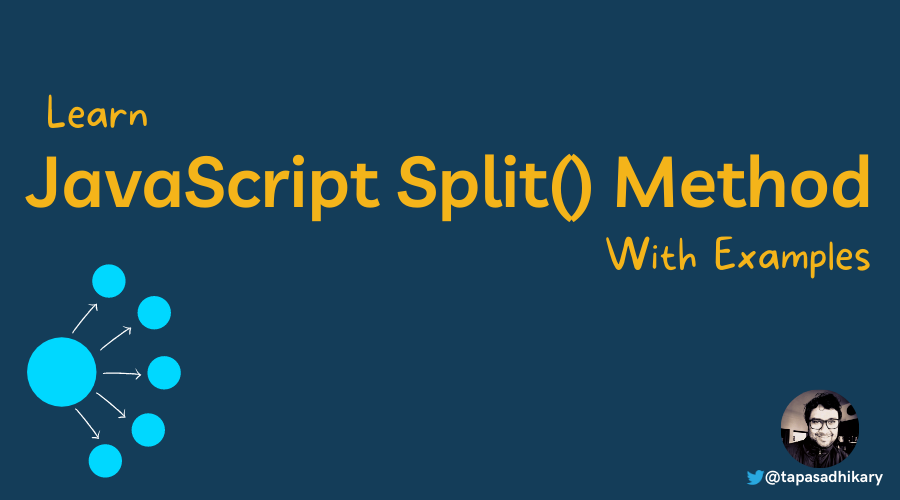
In general, a string
represents a sequence of characters in a programming language.
Permit's await at an example of a string created using a sequence of characters, "Yes, You lot Can DO Information technology!". In JavaScript, nosotros tin can create a string in a couple of ways:
- Using the string literal as a primitive
const msg = "Aye, You Can Do It!";
- Using the
String()
constructor as an object
const msg = new String("Yes, You Can DO It!");
One interesting fact most strings in JavaScript is that we can access the characters in a string using its index. The index of the first character is 0, and information technology increments past 1. So, we tin access each of the characters of a string like this:
let str = "Yep, You Tin can Exercise It!"; panel.log(str[0]); // Y console.log(str[1]); // eastward console.log(str[2]); // due south panel.log(str[iii]); // , console.log(str[10]); // a
The prototype below represents the same thing:
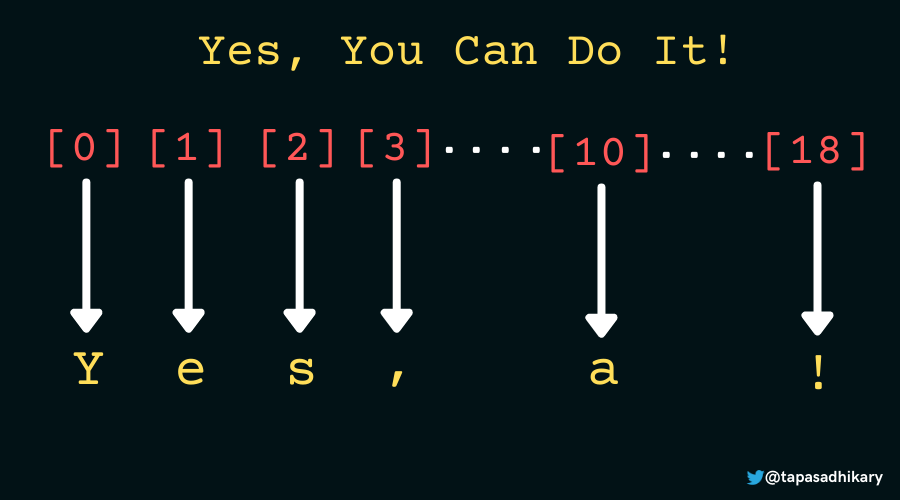
Apart from its ability to access string characters past their indices, JavaScript also provides plenty of utility methods to access characters, have out a part of a cord, and manipulate it.
In this commodity volition learn nigh a handy string method called split()
. I hope you enjoy reading information technology.
The split() Method in JavaScript
The split()
method splits (divides) a string into 2 or more substrings depending on a splitter
(or divider). The splitter can be a single character, another string, or a regular expression.
Later splitting the string into multiple substrings, the split up()
method puts them in an array and returns it. It doesn't make any modifications to the original string.
Let's understand how this works with an example. Here is a string created using string literals:
let message = 'I am a Happy Go lucky Guy';
We can call the carve up()
method on the message
cord. Let's carve up the cord based on the space (' '
) character. Here the infinite character acts as a splitter or divider.
// Split using a space character let arr = message.divide(' '); // The array console.log(arr); // ["I", "am", "a", "Happy", "Get", "lucky", "Guy"] // Admission each of the elements of the array. console.log(arr[0]); // "I" console.log(arr[ane]); // "am" console.log(arr[two]); // "a" console.log(arr[iii]); // "Happy" panel.log(arr[four]); // "Get", panel.log(arr[v]); // "lucky" panel.log(arr[6]); // "Guy"
The primary purpose of the split()
method is to get the chunks you're interested in from a string to use them in any farther utilize cases.
How to Split a String by Each Grapheme
You can split a string past each graphic symbol using an empty string('') every bit the splitter. In the instance below, nosotros split the aforementioned message using an empty string. The result of the split will exist an assortment containing all the characters in the message cord.
console.log(message.split up('')); // ["I", " ", "a", "k", " ", "a", " ", "H", "a", "p", "p", "y", " ", "1000", "o", " ", "l", "u", "c", "k", "y", " ", "G", "u", "y"]
💡 Delight note that splitting an empty string('') using an empty string every bit the splitter returns an empty array. Yous may become this as a question in your upcoming job interviews!
''.split(''); // returns []
How to Dissever a String into One Assortment
You can invoke the carve up()
method on a string without a splitter/divider. This just means the split()
method doesn't have any arguments passed to it.
When you invoke the split()
method on a string without a splitter, it returns an array containing the entire cord.
let bulletin = 'I am a Happy Go lucky Guy'; console.log(bulletin.split()); // returns ["I am a Happy Go lucky Guy"]
💡 Note again, calling the split()
method on an empty string('') without a splitter volition return an assortment with an empty string. It doesn't return an empty array.
Here are two examples so y'all tin can see the difference:
// Returns an empty array ''.split(''); // returns [] // Returns an array with an empty string ''.split() // returns [""]
How to Separate a String Using a Not-matching Character
Commonly, we utilize a splitter that is part of the string we are trying to divide. There could be cases where you may take passed a splitter that is non part of the cord or doesn't match any part of information technology. In that example, the split()
method returns an array with the entire cord as an element.
In the example below, the message string doesn't have a comma (,) character. Let's try to divide the cord using the splitter comma (,).
let message = 'I am a Happy Go lucky Guy'; panel.log(message.dissever(',')); // ["I am a Happy Go lucky Guy"]
💡 Yous should be aware of this as it might help you debug an issue due to a trivial fault like passing the incorrect splitter in the split up()
method.
How to Dissever with a Limit
If you lot idea that the split()
method only takes the splitter equally an optional parameter, let me tell you that there is one more. You tin as well pass the limit
as an optional parameter to the split()
method.
string.split(splitter, limit);
Equally the name indicates, the limit
parameter limits the number of splits. It means the resulting array will only have the number of elements specified by the limit parameter.
In the case below, we dissever a string using a space (' ') every bit a splitter. We as well laissez passer the number 4
as the limit. The split()
method returns an array with merely iv elements, ignoring the residue.
permit message = 'I am a Happy Get lucky Guy'; console.log(message.split(' ', 4)); // ["I", "am", "a", "Happy"]
How to Split up Using Regex
We can also pass a regular expression (regex) as the splitter/divider to the dissever()
method. Let's consider this string to carve up:
let message = 'The sky is blueish. Grass is greenish! Do you know the color of the Deject?';
Let's separate this string at the period (.), exclamation point (!), and the question marking (?). Nosotros can write a regex that identifies when these characters occur. Then we laissez passer the regex to the split()
method and invoke it on the higher up cord.
let sentences = message.carve up(/[.,!,?]/); console.log(sentences);
The output looks like this:

Y'all tin can use the limit
parameter to limit the output to simply the first three elements, similar this:
sentences = message.split(/[.,!,?]/, 3); console.log(sentences);
The output looks like this:
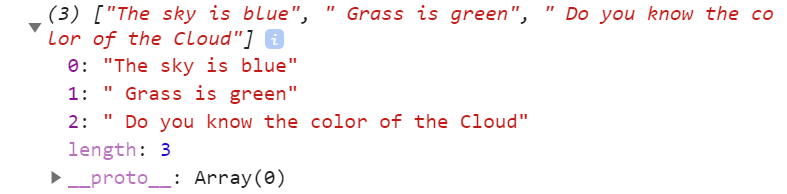
💡 If you want to capture the characters used in the regular expressions in the output array, you need to tweak the regex a fleck. Use parenthesis to capture the matching characters. The updated regex volition be /([.,!,?])/
.
How to Supersede Characters in a String using Split() Method
Yous can solve many interesting problems using the divide()
method combined with other string and assortment methods. Let'due south see i here. Information technology could be a common utilize case to supersede all the occurrences of a graphic symbol in the string with another character.
For example, you may want to create the id
of an HTML element from a proper noun value. The name value may comprise a space (' '), only in HTML, the id value must not contain whatever spaces. We can do this in the post-obit manner:
permit proper name = 'Tapas Adhikary'; let subs = proper noun.dissever(' '); console.log(subs); // ["Tapas", "Adhikary"] let joined = subs.join('-'); console.log(joined); // Tapas-Adhikary
Consider the name has the first name (Tapas) and concluding name (Adhikary) separated by a space. Here we offset separate the proper name using the infinite splitter. It returns an array containing the first and concluding names as elements, that is['Tapas', 'Adhikary']
.
Then nosotros use the array method called join()
to join the elements of the array using the -
character. The join()
method returns a string by joining the chemical element using a character passed as a parameter. Hence we get the final output as Tapas-Adhikary
.
ES6: How to Separate with Array Destructuring
ECMAScript2015 (ES6) introduced a more than innovative way to excerpt an chemical element from an array and assign it to a variable. This smart syntax is known as Array Destructuring
. Nosotros can utilize this with the split()
method to assign the output to a variable hands with less lawmaking.
let proper noun = 'Tapas Adhikary'; let [firstName, lastName] = proper noun.dissever(' '); console.log(firstName, lastName);
Here we split the proper noun using the space graphic symbol equally the splitter. Then nosotros assign the returned values from the array to a couple of variables (the firstName
and lastName
) using the Array Destructuring syntax.
Before We Finish...
👋 Do you want to code and learn forth with me? You can find the same content hither in this YouTube Video. But open up up your favorite code editor and get started.
I hope y'all've found this article insightful, and that it helps you understand JavaScript String's separate()
method more clearly. Please practice the examples multiple times to get a good grip on them. You can find all the lawmaking examples in my GitHub repository.
Allow'due south connect. You will find me active on Twitter (@tapasadhikary). Please feel costless to give a follow.
You may as well like these manufactures:
- The JavaScript Array Handbook – JS Array Methods Explained with Examples
- 10 DevTools tricks to help yous with CSS and UX design
- A practical guide to object destructuring in JavaScript
- 10 trivial yet powerful HTML facts you must know
- x VS Code emmet tips to make y'all more productive
Learn to code for free. freeCodeCamp'due south open source curriculum has helped more than than xl,000 people get jobs as developers. Get started
How To Turn Log Splitter Into Filter Crusher,
Source: https://www.freecodecamp.org/news/javascript-split-how-to-split-a-string-into-an-array-in-js/
Posted by: pagelfarge1999.blogspot.com
0 Response to "How To Turn Log Splitter Into Filter Crusher"
Post a Comment